Graceful Error Handling in React Native: The Power of Error Boundaries
Posted in error-handling, react-error-boundary on September 16, 2023 by Hemanta Sapkota ‐ 3 min read
Imagine using an app when suddenly, it crashes, leaving you facing a blank screen, a mixture of confusion and frustration setting in. As developers, our goal is to prevent these jarring moments. Here's a candid tale from my recent work: I had released a new build to the app store, only to discover a critical oversight. A bug, rooted in an attempt to access an undefined object property, caused a crash that presented users with an unwelcome black screen. This incident served as a stark reminder of the cascading effects a single error can have. It's precisely for such scenarios that error boundaries are essential. They act as a safety net, catching errors in a component tree and preventing them from crashing the entire app. Thanks to error boundaries, we can ensure that an error in one part doesn't compromise the user's experience with the whole application.
1. What is an Error Boundary?
In React Native, an error boundary is a component that gracefully catches JavaScript errors in its child component tree, logs these errors, and displays a fallback UI instead of allowing the entire application to break down. It acts much like a catch {}
block in JavaScript, but for components.
2. Why Do We Need Them?
Errors are inevitable in any application, but the way we handle them defines the user experience. Without error boundaries, a single JavaScript error can bring down the entire app, leading to a complete UI freeze or crash. With error boundaries, we can isolate faulty components, keeping the app mostly functional while providing a more user-friendly response to the unexpected.
3. How to Implement Them
Implementing error boundaries in React Native is straightforward. We utilize the componentDidCatch()
lifecycle method, which catches errors in any component below it in the tree. Here’s a brief on how to create an error boundary component:
export class AppErrorBoundary extends React.Component {
// Initialize state with null values for error and errorInfo
state = { error: null, errorInfo: null };
componentDidCatch(error, errorInfo) {
// Catch errors in any components below and re-render with error message
this.setState({
error: error,
errorInfo: errorInfo,
});
}
render() {
if (this.state.errorInfo) {
// Error path
return (
// Fallback UI
);
}
// Normally, just render children
return this.props.children;
}
}
When an error is caught, the error
and errorInfo
states are set, triggering a re-render to display a fallback UI.
4. Screenshot Demo
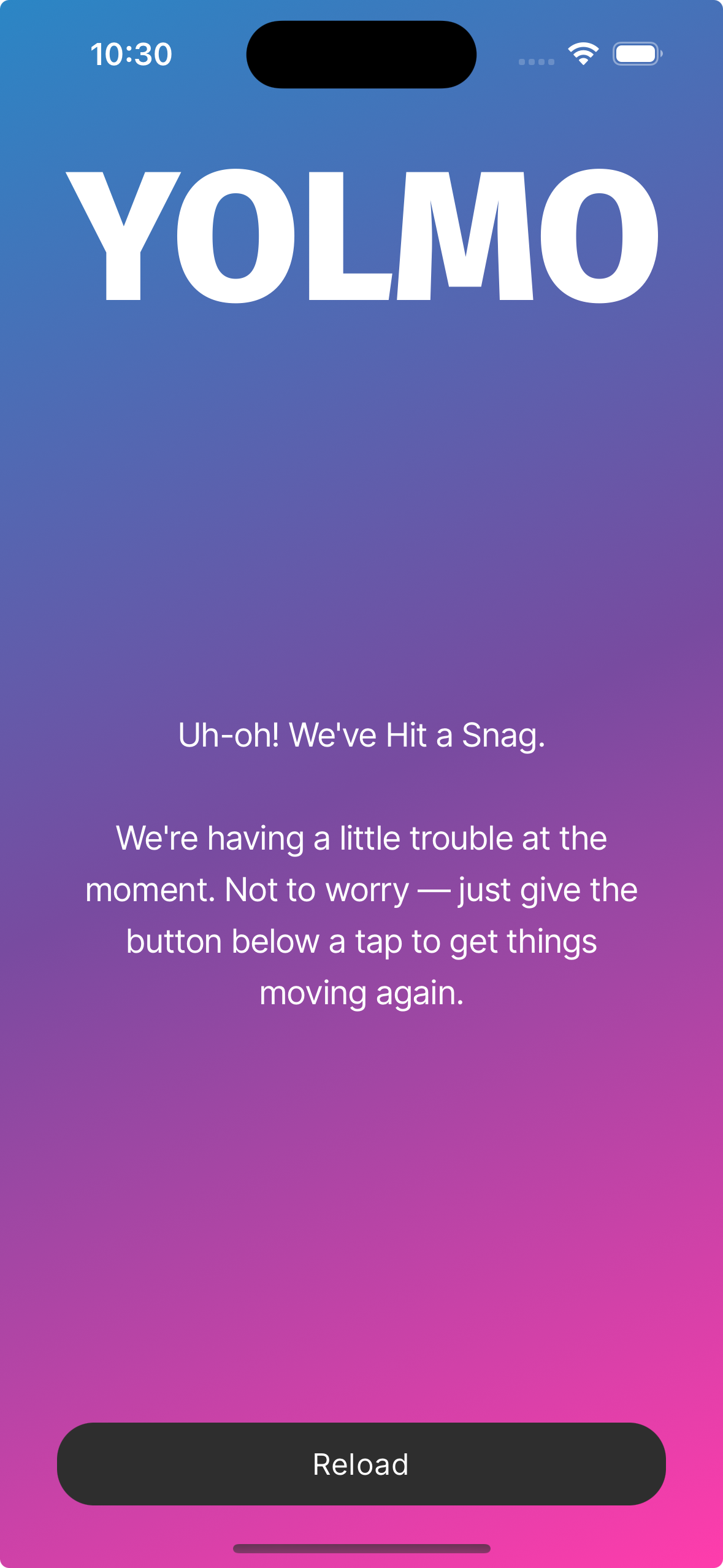
Above is a visual example of what users might see when an error is caught in the Yolmo: Learn to Code app. Notice the friendly notification and the prominent ‘Reload’ button, inviting users to try reloading the application.
5. Conclusion
Error boundaries are essential in any React Native application. They ensure that a single error doesn’t disrupt the entire app, improving overall user experience and application stability. While they are not a silver bullet for all types of errors, they are a critical part of a robust error handling strategy.
Additional Considerations
Remember that error boundaries do not catch errors within:
- Event handlers
- Asynchronous code
- Server-side rendering
- Themselves (the error boundary components)
Code Walkthrough
In the provided code example, AppErrorBoundary
is a class component that defines an error boundary. The componentDidCatch
method sets the error state, which determines if the fallback UI should be displayed. The onRefresh
prop is a function passed from the parent that can reset the app state after an error.
Practical Tips
To maximize the effectiveness of error boundaries:
- Implement centralized error handling services like Sentry.
- Customize the fallback UI to align with your app’s theme and provide clear instructions on what users should do next.
Summary
Integrating error boundaries into your React Native projects can significantly improve your app’s resilience and user satisfaction. It’s a best practice that separates good apps from great ones.
And there you have it, a crash course in maintaining your app’s composure even when the unexpected happens. Implement error boundaries, and let your users navigate with confidence, knowing that a single error won’t ruin their experience.
comments powered by Disqus